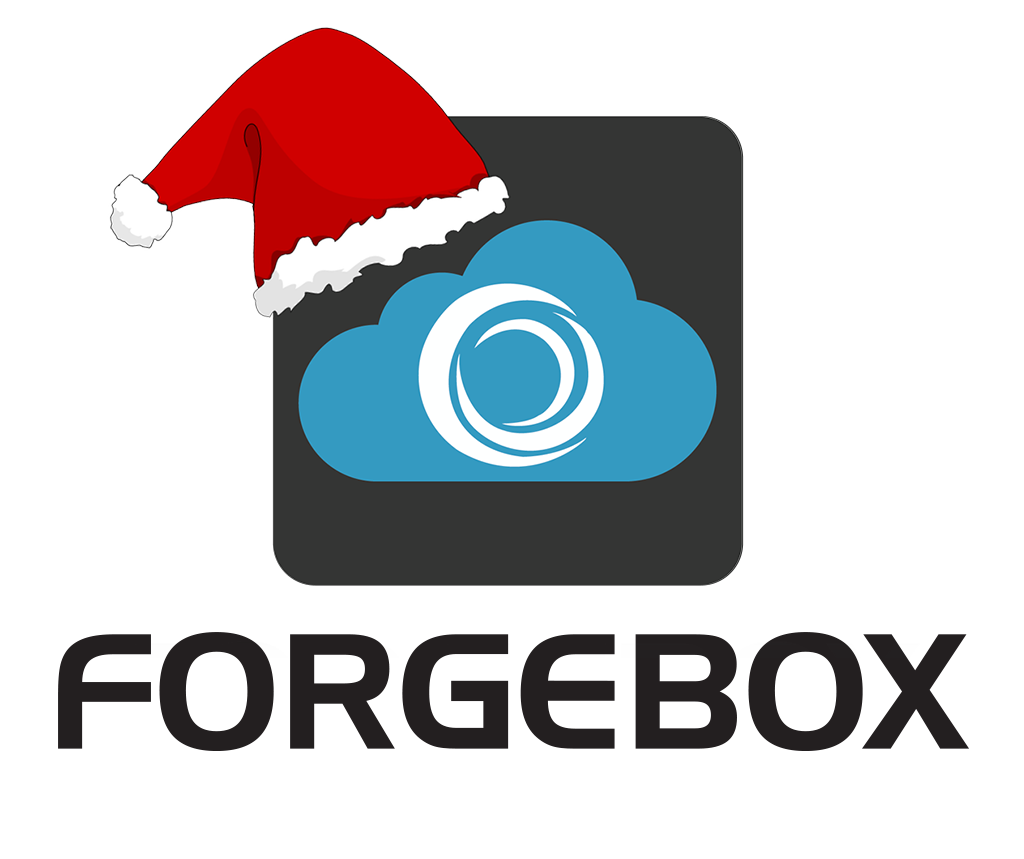
Hyper
Hyper exists to make your life making network requests easier.
Here's the simplest Hyper example:
1 |
|
The HyperResponse
object returned has a few helpers to make it easy to work with requests,
including retrieving the status code, inspecting headers, and deserializing json bodies.
The more complex you get with your HTTP requests, the more Hyper shines. A POST
request looks like so:
1 2 3 4 5 |
"title" = "The Last Jedi" , "episode_id" = 8, "release_date" = "2018-12-15" } ); |
This sends a json
body, by default. Need form fields and a custom header?
1 2 3 4 5 6 7 8 |
var res = hyper .asFormFields() .withHeaders( { "X-Prerelease-Token" = token } ) "title" = "The Last Jedi" , "episode_id" = 8, "release_date" = "2018-12-15" } ); |
While this is a nice syntax improvement over cfhttp
, Hyper was built to solve the
pain points around writing SDK's like GitHub, Stripe, and Twilio. When working with
services like these, there is a handful of boilerplate headers, parameters, or other
values you need to set.
Hyper lets you create clients — partially configured instances that pass on their
defaults to every request made from them. You create a new client with WireBox
(most likely in your config/WireBox.cfc
):
1 2 3 4 5 6 7 8 9 |
map( "GitHubClient" ) .to( "hyper.models.HyperBuilder" ) .asSingleton() .initWith( headers = { "Authorization" = getSetting( "GITHUB_TOKEN" ) } ); |
This client can then be injected and used elsewhere in your application:
1 2 3 4 5 6 7 8 9 |
component { property name= "githubClient" inject= "GithubClient" ; function getRepos() { return githubClient.get( "/user/repos" ); } } |
No more repeating yourself all over the place with the same defaults! And if you need to override a default, just set it as normal. Hyper has a full fluent API to set all the parameters you would need.
So check out the documentation over on ForgeBox and stop rewriting the same cfhttp
code over and over again.
Merry Christmas!
Add Your Comment
(1)
Dec 14, 2017 06:56:06 UTC
by Chris Geirman
Impressive! I recall there being many nitnoid issues with json deserialization in CF. I don't recall specific pain points however. Assuming you know what I mean, have you solved for them?